介绍
简单来说,shape就是用来在xml文件中定义形状,代码解析之后就可以当做Drawable一样使用
官方说明
关于shape定义的drawable
文件位置:res/drawable/filename.xml
编译资源类型:*GradientDrawable*
文件引用:
In Java: R.drawable.filename
In XML: @[package:]drawable/filename
语法:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| <?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape=["rectangle" | "oval" | "line" | "ring"] > <corners android:radius="integer" android:topLeftRadius="integer" android:topRightRadius="integer" android:bottomLeftRadius="integer" android:bottomRightRadius="integer" /> <gradient android:angle="integer" android:centerX="integer" android:centerY="integer" android:centerColor="integer" android:endColor="color" android:gradientRadius="integer" android:startColor="color" android:type=["linear" | "radial" | "sweep"] android:useLevel=["true" | "false"] /> <padding android:left="integer" android:top="integer" android:right="integer" android:bottom="integer" /> <size android:width="integer" android:height="integer" /> <solid android:color="color" /> <stroke android:width="integer" android:color="color" android:dashWidth="integer" android:dashGap="integer" /> </shape>
|
这里只做简单的描述,主要看看使用方式。关于元素的详细说明,请看 shape说明
基本使用
矩形
填充(solid)
1 2 3 4 5 6
| <?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle"> <solid android:color="@color/colorAccent" /> </shape>
|

描边(stroke)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| <?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle">
<solid android:color="@color/colorAccent" />
<stroke android:width="3dp" android:color="@color/colorPrimaryDark" android:dashGap="0dp" android:dashWidth="10dp" /> </shape>
|

圆角(corner)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| <?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle">
<solid android:color="@color/colorAccent" />
<stroke android:width="3dp" android:color="@color/colorPrimaryDark" android:dashGap="4dp" android:dashWidth="10dp" /> <corners android:bottomLeftRadius="60dp" android:radius="30dp" android:topRightRadius="120dp" /> </shape>
|

渐变(gradient):linear
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| <?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle">
<stroke android:width="3dp" android:color="@color/colorPrimaryDark" android:dashGap="4dp" android:dashWidth="10dp" /> <corners android:bottomLeftRadius="60dp" android:radius="30dp" android:topRightRadius="120dp" /> <gradient android:angle="45" android:centerColor="@color/stone" android:endColor="@color/pink" android:startColor="@color/yellow" />
</shape>
|
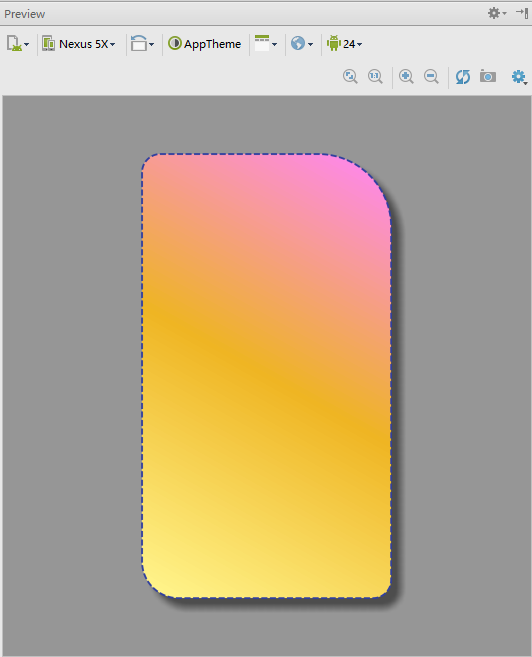
渐变(gradient):radial
1 2 3 4 5 6 7
| <gradient android:angle="90" android:startColor="@color/colorPrimary" android:centerColor="@color/pink" android:endColor="@color/yellow" android:gradientRadius="400dp" android:type="radial"/>
|

渐变(gradient):sweep
1 2 3 4 5 6
| <gradient android:startColor="@color/colorPrimary" android:centerColor="@color/pink" android:endColor="@color/yellow" android:gradientRadius="400dp" android:type="sweep"/>
|

圆形
正圆
1 2 3 4 5 6 7 8 9 10 11
| <?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="oval"> <gradient android:centerColor="@color/pink" android:endColor="@color/yellow" android:startColor="@color/colorPrimary" /> <size android:width="400dp" android:height="400dp" /> </shape>
|
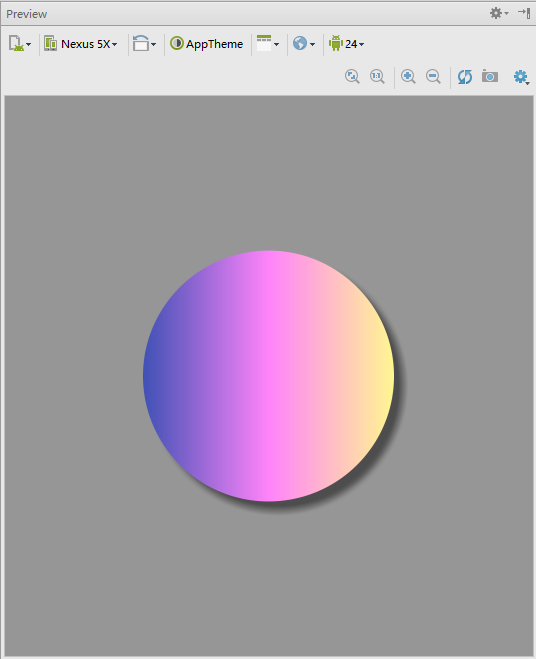
椭圆
1 2 3 4 5 6 7 8 9
| <?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="oval"> <gradient android:angle="90" android:centerColor="@color/pink" android:endColor="@color/yellow" android:startColor="@color/colorPrimary" /> </shape>
|
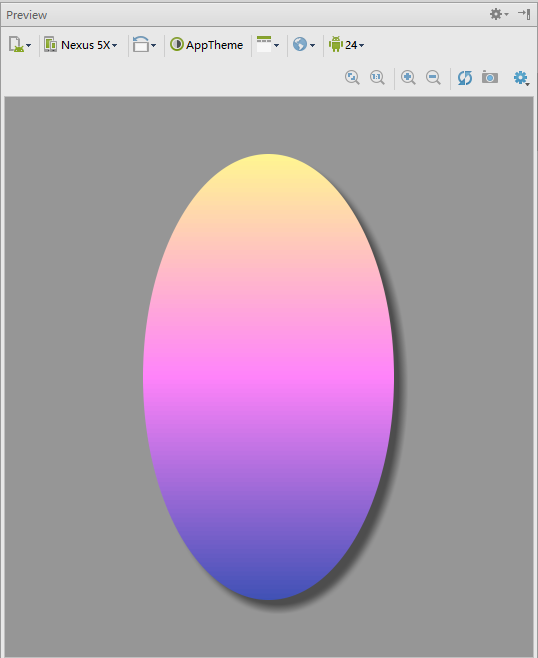
线条
1 2 3 4 5 6 7
| <?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="line"> <stroke android:width="9dp" android:color="@color/pink" /> </shape>
|
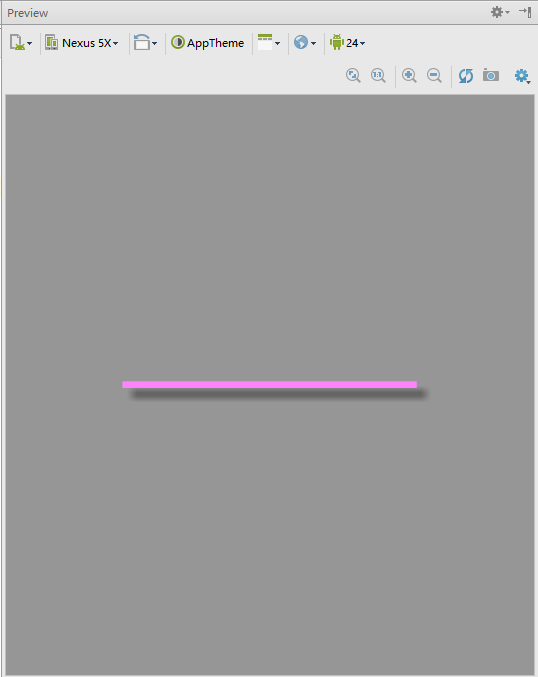
环形
1 2 3 4 5 6 7 8 9 10 11
| <?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="ring" android:innerRadius="100dp" android:thickness="50dp" android:useLevel="false" > <gradient android:startColor="@color/colorAccent" android:endColor="@color/yellow" android:centerColor="@color/pink"/> </shape>
|
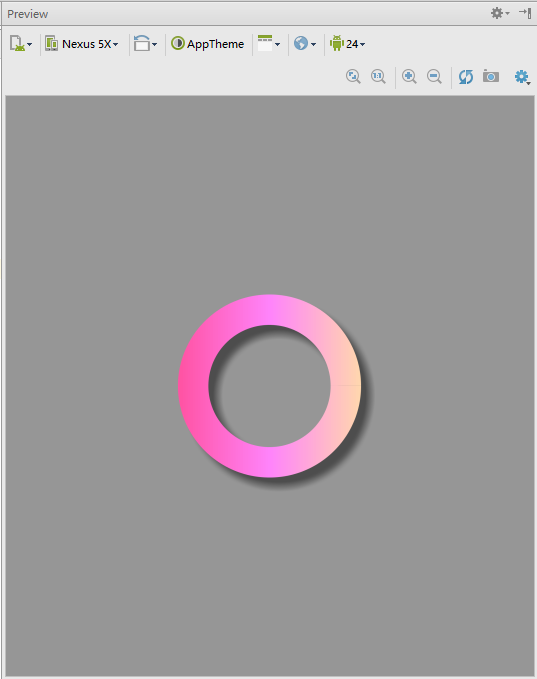
android:useLevel=”false”这个属性值一定要设置成false,根据google官网上的解释:

以下属性只能在 android:shape="ring"
的时候使用:
属性 |
意义 |
android:innerRadius |
尺寸,内环的半径 |
android:thickness |
尺寸,环的厚度 |
android:innerRadiusRatio |
浮点型,以环的宽度比率来表示内环的径, 例如,如果android:innerRadiusRatio=5,表示内环半径等于的宽度除以5,这个值是可以被android:innerRadius的值覆盖,默认9. |
android:thicknessRatio |
浮点型,以环的宽度比率来表示环的度,例如,如果android:thicknessRatio=2, 那么环的厚度就等于的宽度除以2。这个值是可以被android:thickness覆盖的,默认值是3. |
android:useLevel |
boolean值,如果当做LevelListDrawable使用时值为true,否则为false.这个值一般false,否则你的环可能不会出现 |
其他说明
这些自己定义的shape为根节点的drawable xml文件,可以用来当成背景使用在Button,TextView等视图上,同时由于可以设置size大小,也可以用来制作简单的图标等。总而言之,每个细小的东西,都有挖掘的价值,感觉这里面还有一些东西我没有注意到,还要好好的看一下文档。
最后,google镜像网站,xsoftlab,当然有条件的建议使用Google官网。